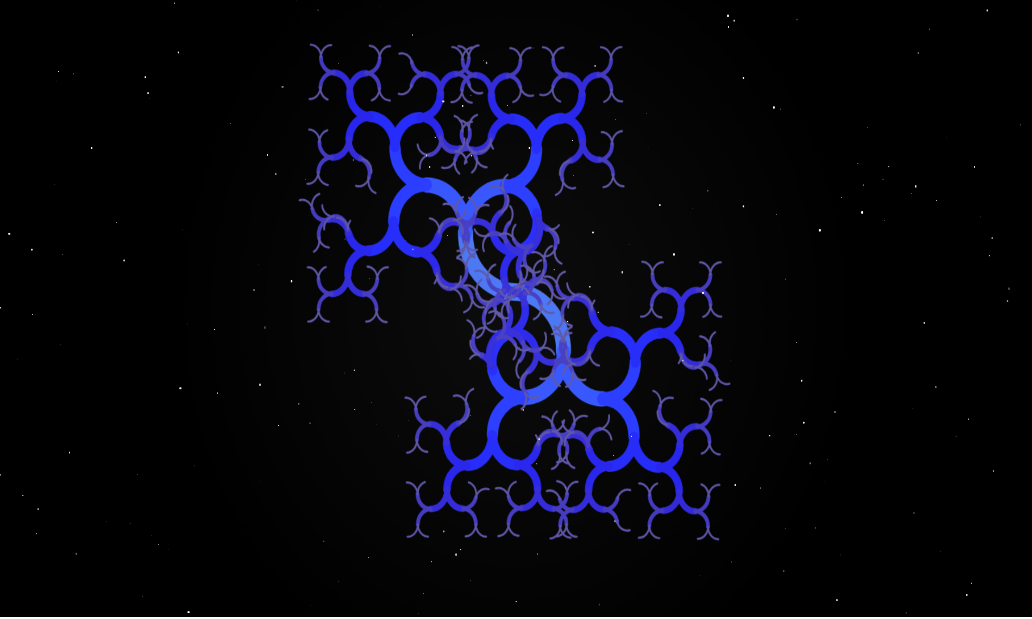
Create a Stunning Starry Space Animation with HTML, CSS, and JavaScript
Ever wanted to bring the beauty of a starry night sky to your website? With just a few lines of HTML, CSS, and JavaScript, you can create a mesmerizing space animation featuring twinkling stars and a smooth, immersive effect.
In this guide, we’ll go step by step to create a stunning starry background effect that can be used for website backgrounds, hero sections, or even interactive projects.
Why Create a Starry Space Animation?
A starry animation is not just visually appealing; it also adds a touch of depth and elegance to a website. Whether you’re working on a sci-fi project, a portfolio, or a creative landing page, this effect can significantly enhance user experience.
Here’s what we’ll achieve:
✅ A canvas-based starry background
✅ Randomly positioned stars with varying sizes
✅ Twinkling and moving effects for realism
✅ Lightweight and optimized for performance
Step 1: Setting Up the HTML Structure
We’ll start with a simple HTML file. The only thing we need is a element, which will serve as our drawing board for the stars.
Step 2: Styling the Canvas with CSS
To ensure our animation covers the full screen and looks clean, we’ll apply some CSS styles.
Step 3: Adding Stars with JavaScript
Now, let’s use JavaScript to draw stars and make them twinkle. We’ll use the Canvas API
to generate randomly positioned stars and animate them.
Step 4: Making It Responsive
To ensure the canvas resizes dynamically when the window size changes, add this code:
Bonus: Adding a Shooting Star Effect
To add a shooting star effect, we can introduce another class that creates a fast-moving streak across the screen at random intervals.
Final Thoughts
With just a few lines of HTML, CSS, and JavaScript, we’ve built an eye-catching starry space animation that works beautifully as a background. You can tweak the number of stars, speed, colors, and effects to fit your project.
Ways to Enhance the Animation:
? Add a parallax effect by moving stars at different speeds
? Introduce a gradient background for more depth
? Make the stars react to mouse movements
? Use WebGL for an even smoother experience
Try experimenting with different settings and bring your own creativity to the project. ?✨
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<!-- Space background -->
<div class="space">
<div class="stars"></div>
</div>
<!-- Light rays effect -->
<div class="light-rays"></div>
</body>
</html>
CSS Code
/* General Styles */ body { margin: 0; overflow: hidden; background: black; position: relative; } /* Starry Background */ .stars { width: 100%; height: 100%; position: absolute; animation: moveStars 30s linear infinite; } @keyframes moveStars { from { transform: translateY(0); } to { transform: translateY(-100vh); } } /* Light Rays Effect */ .light-rays { position: absolute; width: 100%; height: 100vh; background: radial-gradient(circle, rgba(255, 255, 255, 0.1) 0%, transparent 60%); opacity: 0.3; animation: pulse 5s infinite alternate ease-in-out; } @keyframes pulse { from { opacity: 0.2; } to { opacity: 0.5; } } /* Creating multiple stars */ .star { position: absolute; background: white; border-radius: 50%; width: 2px; height: 2px; opacity: 0.8; }
JavaScript Code
// Creating stars dynamically function createStars() { const space = document.querySelector(".space"); for (let i = 0; i < 200; i++) { let star = document.createElement("div"); star.classList.add("star"); let x = Math.random() * window.innerWidth; let y = Math.random() * window.innerHeight; let size = Math.random() * 3; let duration = Math.random() * 5 + 2; star.style.left = `${x}px`; star.style.top = `${y}px`; star.style.width = `${size}px`; star.style.height = `${size}px`; star.style.animation = `twinkle ${duration}s infinite alternate`; space.appendChild(star); } } createStars(); // Twinkling animation const style = document.createElement("style"); style.innerHTML = ` @keyframes twinkle { 0% { opacity: 0.2; } 100% { opacity: 1; } } `; document.head.appendChild(style); "use strict"; // Paul Slaymaker, paul25882@gmail.com const body=document.getElementsByTagName("body").item(0); body.style.background="#000"; const TP=2*Math.PI; const CSIZE=400; const ctx=(()=>{ let d=document.createElement("div"); d.style.textAlign="center"; body.append(d); let c=document.createElement("canvas"); c.width=c.height=2*CSIZE; d.append(c); return c.getContext("2d"); })(); ctx.translate(CSIZE,CSIZE); ctx.lineCap="round"; onresize=()=>{ let D=Math.min(window.innerWidth,window.innerHeight)-40; ctx.canvas.style.width=ctx.canvas.style.height=D+"px"; } const getRandomInt=(min,max,low)=>{ if (low) return Math.floor(Math.random()*Math.random()*(max-min))+min; else return Math.floor(Math.random()*(max-min))+min; } function Color() { const CBASE=144; const CT=255-CBASE; this.getRGB=(ct)=>{ let red=Math.round(CBASE+CT*(Math.cos(this.RK2+ct/this.RK1))); let grn=Math.round(CBASE+CT*(Math.cos(this.GK2+ct/this.GK1))); let blu=Math.round(CBASE+CT*(Math.cos(this.BK2+ct/this.BK1))); return "rgb("+red+","+grn+","+blu+")"; } this.randomize=()=>{ this.RK1=360+360*Math.random(); this.GK1=360+360*Math.random(); this.BK1=360+360*Math.random(); this.RK2=TP*Math.random(); this.GK2=TP*Math.random(); this.BK2=TP*Math.random(); } this.randomize(); } var color=new Color(); var stopped=true; var start=()=>{ if (stopped) { stopped=false; requestAnimationFrame(animate); } else { stopped=true; } } body.addEventListener("click", start, false); var dur=2000; var tt=getRandomInt(0,1000); var pause=0; var trans=false; var animate=(ts)=>{ if (stopped) return; tt++; draw(); requestAnimationFrame(animate); } var KF=Math.random(); var Circle=function() { this.dir=false; this.r=80; this.randomize=()=>{ this.ka=0; //TP*Math.random(); this.ka2=200; } this.randomize(); this.setRA=()=>{ this.a2=TP/4+1.57*(1+Math.sin(tt/this.ka2))/2; if (this.dir) this.a2=-this.a2; } this.setPath2=()=>{ if (this.p) { if (this.cont) { this.a=TP/2+this.p.a-this.p.a2; this.x=this.p.x+(this.p.r-this.r)*Math.cos(this.p.a-this.p.a2); this.y=this.p.y+(this.p.r-this.r)*Math.sin(this.p.a-this.p.a2); } else { this.a=this.p.a-this.p.a2; this.x=this.p.x+(this.p.r+this.r)*Math.cos(this.p.a-this.p.a2); this.y=this.p.y+(this.p.r+this.r)*Math.sin(this.p.a-this.p.a2); } } else { this.x=this.r*Math.cos(this.a); this.y=this.r*Math.sin(this.a); } this.path=new Path2D(); this.path.arc(this.x,this.y,this.r,TP/2+this.a,this.a-this.a2,this.dir); } } onresize(); var ca=[]; var reset=()=>{ ca=[new Circle()]; ca[0].p=false; ca[0].a=0; //TP*Math.random(); ca[0].setRA(); ca[0].x=ca[0].r*Math.cos(ca[0].a); ca[0].y=ca[0].r*Math.sin(ca[0].a); } reset(); var addCircle=(c)=>{ let c2=new Circle(); c2.a=c.a-c.a2; c2.dir=!c.dir; c2.p=c; c2.cont=false; c2.r=c.r*0.8; if (Math.random()<KF) c2.ka2=c.ka2*0.8; ca.push(c2); let c3=new Circle(); c3.a=TP/2+c.a-c.a2; c3.dir=c.dir; c3.p=c; c3.cont=true; c3.r=c.r*0.8; if (Math.random()<1-KF) c3.ka2=c.ka2*0.8; ca.push(c3); } const dmx=new DOMMatrix([-1,0,0,1,0,0]); const dmy=new DOMMatrix([1,0,0,-1,0,0]); const dmxy=new DOMMatrix([-1,0,0,-1,0,0]); //const dmq=new DOMMatrix([0,1,-1,0,0,0]); const getXYPath=(spath)=>{ this.level=1; let p=new Path2D(spath); p.addPath(p,dmxy); return p; } var draw=()=>{ ca[0].a=tt/1000; for (let i=0; i<ca.length; i++) ca[i].setRA(); for (let i=0; i<ca.length; i++) ca[i].setPath2(); let pa=new Array(8); for (let i=0; i<pa.length; i++) pa[i]=new Path2D(); for (let i=0; i<ca.length; i++) { if (i==0) pa[0].addPath(ca[i].path); else if (i<3) pa[1].addPath(ca[i].path); else if (i<7) pa[2].addPath(ca[i].path); else if (i<15) pa[3].addPath(ca[i].path); else if (i<31) pa[4].addPath(ca[i].path); else if (i<63) pa[5].addPath(ca[i].path); else if (i<127) pa[6].addPath(ca[i].path); else pa[7].addPath(ca[i].path); } ctx.clearRect(-CSIZE,-CSIZE,2*CSIZE,2*CSIZE); for (let i=0; i<pa.length; i++) { let pth=getXYPath(pa[i]); ctx.strokeStyle=color.getRGB(tt-180*i); ctx.lineWidth=Math.max(3, 24-3*i); ctx.stroke(pth); } } for (let i=0; i<127; i++) addCircle(ca[i]); start();
PHP Code
NO Need
? FAQ for Better Google Ranking ?
1️⃣ How do I create a starry night animation using JavaScript? ✨
To create a starry night effect, use the HTML5 element with JavaScript. ? Generate random stars, animate them using the
requestAnimationFrame()
function, and update their opacity for a twinkling effect. ?
2️⃣ Can I use this space animation as a website background? ?️
Yes! ? The starry space animation can be used as a dynamic background by placing the
behind other webpage elements. ? It provides a visually appealing effect for portfolios, landing pages, and creative websites. ?
3️⃣ How do I make the stars twinkle in my animation? ?
To make stars twinkle, randomly change their opacity values over time in small increments. ? This simulates the flickering effect of real stars in the night sky. ✨
4️⃣ Is this animation lightweight and mobile-friendly? ?
Yes! ✅ The animation is optimized for performance by using a lightweight Canvas API
approach. ⚡ Additionally, it includes a resize event listener to ensure it adapts to different screen sizes. ?
5️⃣ How can I add a shooting star effect to the animation? ?
You can create a shooting star effect by drawing moving streaks with decreasing opacity. ? Use a separate JavaScript class for shooting stars and animate them with smooth motion across the screen. ?
6️⃣ Will this animation slow down my website? ?️
No! ? When properly optimized, the animation runs efficiently. You can control the number of stars and adjust performance settings to balance visual effects and page speed. ⚡?
7️⃣ How can I improve the Google ranking of my animation page? ?
To rank higher on Google:
✅ Use SEO-friendly meta tags ?️
✅ Implement structured FAQ schema ?
✅ Optimize for fast page load speed ⚡
✅ Add internal links ? & external links to authority sources ?
✅ Embed a video tutorial ? for better engagement
? Follow these tips, and your animation page will rank higher on Google in no time! ??
Copy & Share
Feel free to use this source code on your projects, share it with others, and leave feedback in the comments section. If you liked this free script, stay tuned for more creative coding tutorials!
Enjoy coding and bring the universe to your website!